Example-1: Write a MATLAB program to find the Fourier transform of the rectangular
pulse.
x(t) = u(t + 1) − u(t − 1)
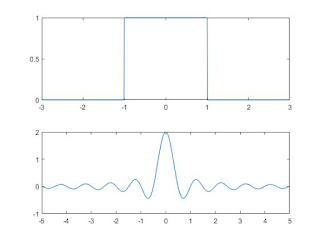
clc; clear all; close all;
tmin=-3;tmax=3;
dt=0.01;t=tmin:dt:tmax;
%*** Signal x(t) ****
xt=1.*(t>=-1&t<=1);
%**** CTFT X(f) ******
k=0;
for f=-5:.01:5
k=k+1;
X(k)=trapz(t,xt.*exp(-j*2*pi*f*t));
end
f=-5:.01:5;
subplot(211);
plot(t,xt);
subplot(212);
plot(f,X);
Example-2: Write a MATLAB program to find the Fourier transform of x(t) = sin(40πt).
Solution:
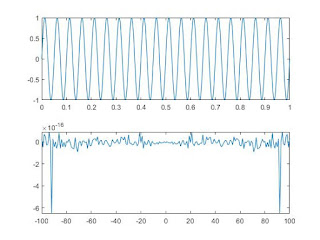
tmin=0;tmax=1;
Ts=0.0002;t=tmin:Ts:tmax;
xt=sin(2*pi*20*t);
k=0;
for f=-100:1:100
k=k+1;
X(k)=trapz(t,xt.*exp(-j*2*pi*f*t));
end
f=-100:100;
subplot(211);
plot(t,xt);
subplot(212);
plot(f,abs(X))
Example-3 Write a MATLAB program to find the Fourier transform of x(t) = cos(40πt).
Solution:
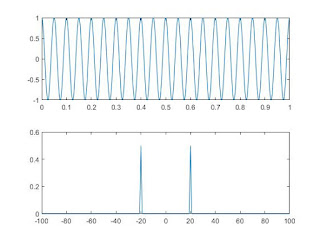
tmin=0;tmax=1;
Ts=0.0002;t=tmin:Ts:tmax;
xt=cos(2*pi*20*t);
k=0;
for f=-100:1:100
k=k+1;
X(k)=trapz(t,xt.*exp(-j*2*pi*f*t));
end
f=-100:100;
subplot(211);
plot(t,xt);
subplot(212);
plot(f,abs(X))
Similarly, for any other continuous time signal, CTFT can be found in MATLAB. The corresponding plots are also given in above Figures.
Comments
Post a Comment